Object detection: Reduced resolution¶
Warning: Runtimes can be several hours even on clusters.
We compared the performance of models from FaceBook’s Detectron project and YOLOv3 model from Joseph Redmon, when different error sources were added. The models from FaceBook’s Detectron project were FasterRCNN, MaskRCNN and RetinaNet.
[1]:
import re
from abc import ABC, abstractmethod
import matplotlib.pyplot as plt
from PIL import Image
from dpemu import runner
from dpemu.dataset_utils import load_coco_val_2017
from dpemu.filters.image import Resolution
from dpemu.ml_utils import run_ml_module_using_cli, load_yolov3
from dpemu.nodes import Array, Series
from dpemu.plotting_utils import print_results_by_model, visualize_scores
from dpemu.utils import get_project_root
We used 118 287 jpg images (COCO train2017) as the train set and 5000 images (COCO val2017) as the test set to calculate the mAP-50 scores.
[2]:
def get_data():
imgs, _, _, img_filenames = load_coco_val_2017()
return imgs, img_filenames
[3]:
def get_err_root_node():
err_node = Array()
err_root_node = Series(err_node)
err_node.addfilter(Resolution("k"))
return err_root_node
Examples from run_yolo_example.py¶
k: 1¶
k: 2¶
k: 3¶
k: 4¶
[4]:
def get_err_params_list():
return [{"k": k} for k in range(1, 5)]
[5]:
class Preprocessor:
def run(self, _, imgs, params):
img_filenames = params["img_filenames"]
for i, img_arr in enumerate(imgs):
img = Image.fromarray(img_arr)
path_to_img = f"{get_project_root()}/tmp/val2017/" + img_filenames[i]
img.save(path_to_img, "jpeg", quality=100)
return None, imgs, {}
Detectron’s model zoo had pretrained weights for FasterRCNN, MaskRCNN and RetinaNet. YOLOv3’s weights were trained by us, using the Kale cluster of University of Helsinki. The training took approximately five days when two NVIDIA Tesla V100 GPUs were used.
[6]:
class YOLOv3Model:
def run(self, _, imgs, params):
path_to_yolov3_weights, path_to_yolov3_cfg = load_yolov3()
cline = f"{get_project_root()}/libs/darknet/darknet detector map {get_project_root()}/data/coco.data \
{path_to_yolov3_cfg} {path_to_yolov3_weights}"
out = run_ml_module_using_cli(cline, show_stdout=False)
match = re.search(r"\(mAP@0.50\) = (\d+\.\d+)", out)
return {"mAP-50": round(float(match.group(1)), 3)}
class AbstractDetectronModel(ABC):
def run(self, _, imgs, params):
path_to_cfg = self.get_path_to_cfg()
url_to_weights = self.get_url_to_weights()
cline = f"""{get_project_root()}/libs/Detectron/tools/test_net.py \
--cfg {path_to_cfg} \
TEST.WEIGHTS {url_to_weights} \
NUM_GPUS 1 \
TEST.DATASETS '("coco_2017_val",)' \
MODEL.MASK_ON False \
OUTPUT_DIR {get_project_root()}/tmp \
DOWNLOAD_CACHE {get_project_root()}/tmp"""
out = run_ml_module_using_cli(cline, show_stdout=False)
match = re.search(r"IoU=0.50 \| area= all \| maxDets=100 ] = (\d+\.\d+)", out)
return {"mAP-50": round(float(match.group(1)), 3)}
@abstractmethod
def get_path_to_cfg(self):
pass
@abstractmethod
def get_url_to_weights(self):
pass
class FasterRCNNModel(AbstractDetectronModel):
def get_path_to_cfg(self):
return f"{get_project_root()}/libs/Detectron/configs/12_2017_baselines/e2e_faster_rcnn_X-101-64x4d-FPN_1x.yaml"
def get_url_to_weights(self):
return (
"https://dl.fbaipublicfiles.com/detectron/35858015/12_2017_baselines/"
"e2e_faster_rcnn_X-101-64x4d-FPN_1x.yaml.01_40_54.1xc565DE/output/train/"
"coco_2014_train%3Acoco_2014_valminusminival/generalized_rcnn/model_final.pkl"
)
class MaskRCNNModel(AbstractDetectronModel):
def get_path_to_cfg(self):
return f"{get_project_root()}/libs/Detectron/configs/12_2017_baselines/e2e_mask_rcnn_X-101-64x4d-FPN_1x.yaml"
def get_url_to_weights(self):
return (
"https://dl.fbaipublicfiles.com/detectron/36494496/12_2017_baselines/"
"e2e_mask_rcnn_X-101-64x4d-FPN_1x.yaml.07_50_11.fkwVtEvg/output/train/"
"coco_2014_train%3Acoco_2014_valminusminival/generalized_rcnn/model_final.pkl"
)
class RetinaNetModel(AbstractDetectronModel):
def get_path_to_cfg(self):
return f"{get_project_root()}/libs/Detectron/configs/12_2017_baselines/retinanet_X-101-64x4d-FPN_1x.yaml"
def get_url_to_weights(self):
return (
"https://dl.fbaipublicfiles.com/detectron/36768875/12_2017_baselines/"
"retinanet_X-101-64x4d-FPN_1x.yaml.08_34_37.FSXgMpzP/output/train/"
"coco_2014_train%3Acoco_2014_valminusminival/retinanet/model_final.pkl"
)
[7]:
def get_model_params_dict_list():
return [
{"model": FasterRCNNModel, "params_list": [{}]},
{"model": MaskRCNNModel, "params_list": [{}]},
{"model": RetinaNetModel, "params_list": [{}]},
{"model": YOLOv3Model, "params_list": [{}]},
]
[8]:
def visualize(df):
visualize_scores(
df,
score_names=["mAP-50"],
is_higher_score_better=[True],
err_param_name="k",
title="Object detection with reduced resolution"
)
plt.show()
[9]:
def main():
imgs, img_filenames = get_data()
df = runner.run(
train_data=None,
test_data=imgs,
preproc=Preprocessor,
preproc_params={"img_filenames": img_filenames},
err_root_node=get_err_root_node(),
err_params_list=get_err_params_list(),
model_params_dict_list=get_model_params_dict_list(),
n_processes=1
)
print_results_by_model(df)
visualize(df)
[10]:
main()
loading annotations into memory...
Done (t=0.52s)
creating index...
index created!
0%| | 0/4 [00:00<?, ?it/s]
25%|██▌ | 1/4 [48:04<2:24:14, 2884.76s/it]
50%|█████ | 2/4 [1:35:50<1:35:58, 2879.18s/it]
75%|███████▌ | 3/4 [2:23:16<47:48, 2868.95s/it]
100%|██████████| 4/4 [3:09:35<00:00, 2842.14s/it]
FasterRCNN #1
mAP-50 | k | time_err | time_pre | time_mod | |
---|---|---|---|---|---|
0 | 0.636 | 1 | 43.079 | 188.690 | 778.357 |
1 | 0.448 | 2 | 43.463 | 189.020 | 773.277 |
2 | 0.192 | 3 | 43.073 | 197.176 | 763.480 |
3 | 0.070 | 4 | 43.302 | 151.681 | 758.630 |
MaskRCNN #1
mAP-50 | k | time_err | time_pre | time_mod | |
---|---|---|---|---|---|
0 | 0.643 | 1 | 43.079 | 188.690 | 773.331 |
1 | 0.448 | 2 | 43.463 | 189.020 | 771.375 |
2 | 0.188 | 3 | 43.073 | 197.176 | 759.624 |
3 | 0.084 | 4 | 43.302 | 151.681 | 758.115 |
RetinaNet #1
mAP-50 | k | time_err | time_pre | time_mod | |
---|---|---|---|---|---|
0 | 0.594 | 1 | 43.079 | 188.690 | 1007.471 |
1 | 0.450 | 2 | 43.463 | 189.020 | 1003.882 |
2 | 0.231 | 3 | 43.073 | 197.176 | 996.645 |
3 | 0.112 | 4 | 43.302 | 151.681 | 982.990 |
YOLOv3 #1
mAP-50 | k | time_err | time_pre | time_mod | |
---|---|---|---|---|---|
0 | 0.556 | 1 | 43.079 | 188.690 | 89.957 |
1 | 0.422 | 2 | 43.463 | 189.020 | 81.409 |
2 | 0.109 | 3 | 43.073 | 197.176 | 79.648 |
3 | 0.023 | 4 | 43.302 | 151.681 | 79.119 |
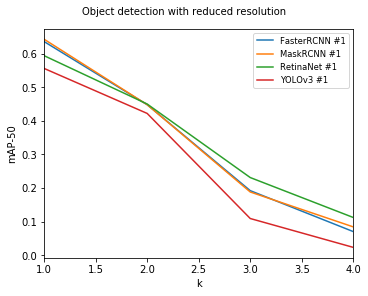
The notebook for this case study can be found here.